In Java programming language, all source code is written first in plain text files ending with the .java extension. Those source files are then compiled into .class files by the java compiler. A .class file doesn't contain code that is native to your processor, it instead contains bytecodes. Bytecode is the machine language of Java Virtual Machine(Java VM). The Java launcher tool then runs your program with an instance of the Java Virtual Machine.
Home » Archives for September 2013
C# - Operators and Expressions

For example:
int number1, number2, sum;
number1 = 10;
number2 = 20;
sum = number1 + number2;
In the last statement, two variables using the + symbol. The + is a operator also there are many operators in c#.
Data Types and Variables
A data type specifies whether or not a variable can store a number, a single character or text. Variables are names given to the memory location for storing data. Data Types are used to specify the types of values related to variables.
How To Use TinyURL API In VB.NET

Thinkspace Seeks To Launch Software Development To High Schools Worldwide

How To Make Awesome Collages
in
drag and drop images,
picturebox,
pictures,
review,
software
- By
Mohamed Shimran
- on 9/09/2013
- No comments
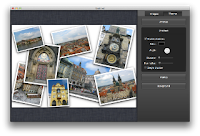
Snippet : Using Regular Expressions in Java
This snippet helps you to use Regular Expression(Regex) in Java.
Snippet : Get ASCII Value For Every Characters
This snippet helps you to get the ASCII value for every characters.
How To Execute Shell Commands and Print Results in Java
in
bash script -eq,
bash script -ne,
best way to learn java,
execute shell commands,
execute shell commands java,
get runtime,
host runtime,
java,
java learning,
oracle sql plsql,
print results,
tutorial
- By
Mohamed Shimran
- on 9/09/2013
- No comments
There are many ways to execute shell commands and print results in java I guess, the famous and the most used way is Runtime.getRuntime().exec you can find a good explanation for that from here. Coming to the point i am using the same way but little different! I use BufferedReader,InputStreamReader for simplicity and I can't make it much advanced. On the main event I have created a String where you put your command with the second event it executes the command and prints the result before I start I would like to give some credits to luismcosta.
Easy-Run : Add, Edit and Remove Computer Startup Items
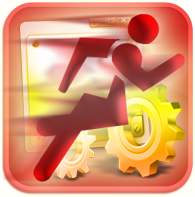
Snippet : Javascript onmouseover and onmouseout
This snippet helps you to make buttons etc.. I have been using this for Download Buttons.
Jarvis : an Artificial Intelligence Operating System
How To Make A Port Scanner in PHP
Port scanners are created to find opened/closed ports in a host, you can get the same results in this Port Scanner coded in php by the way I wouldn't take all the credits to this because i am a php beginner so I had to make this from scratch also there are many more open source advanced Port Scanners. You can also easily add Ports if you need to.
Snippet : Base64 Encode and Base64 Decode in PHP
This snippet helps you to encode and decode your text with base64.
Base64 is a group of similar encoding schemes that represent binary data in an ASCII string format by translating it into a radix-64 representation. The Base64 term originates from a specific MIME content transfer encoding. - Wikipedia
Snippet : TinyURL API in PHP
This snippet helps you to shorten your URLs with TinyURL API(Function with usage)
- <?php
- function TinyURL($url){
- return file_get_contents('http://tinyurl.com/api-create.php?url='.$url);
- }
- echo TinyURL('http://www.ultimateprogrammingtutorials.info');
- ?>
You need to replace http://www.ultimateprogrammingtutorials.info with the URL you want to shorten
Snippet : HTTP Redirection In PHP
This snippet helps you to redirect to another website/webpage.
- <?php
- header('Location: http://www.example.com');
- ?>
Replace http://www.example.com with your website URL/webpage URL
Snippet : Detect HTTP User Agent in PHP
The code below helps you to detect the HTTP user agent I mean the browser version.
- <?php
- $useragent = $_SERVER['HTTP_USER_AGENT'];
- echo "<b>User Agent </b>: ".$useragent;
- ?>
Return
User Agent : Mozilla/5.0 (Windows NT 6.1) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/29.0.1547.62 Safari/537.36
User Agent : Mozilla/5.0 (Windows NT 6.1) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/29.0.1547.62 Safari/537.36
Down For Everyone Or Just Me in VB.NET

Required components
- One Button
- One TextBox
Coding :
Add these codes to Button click_event & don't forget to add the namespace Imports System.Net.'Imports System.Net 'defining strin as a string & setting it to download strings from the website & the URL Dim strin As String = New WebClient().DownloadString(("http://downforeveryoneorjustme.com/" + TextBox1.Text)) 'checking string If strin.Contains("It's just you.") Then 'return MsgBox(TextBox1.Text + " is up", MessageBoxIcon.Information, MessageBoxButtons.OK) 'checking string ElseIf strin.Contains("It's not just you!") Then 'return MsgBox(TextBox1.Text + " is down", MessageBoxIcon.Information, MessageBoxButtons.OK) 'checking string ElseIf strin.Contains("Huh?") Then 'return MsgBox("Invalid website", MessageBoxIcon.Error, MessageBoxButtons.OK) End IfNow to check you can just enter the URL you want to check in TextBox and click on the Button you added.